Test your memory with this game!
LEDs will blink in a pattern that you have to remember and repeat. If you get it right, the game gets more and more challenging.
Materials
- 1 Arduino Uno board
- 1 Education Shield
- 1 breadboard
- 1 TinkerKit button
- 1 potentiometer
- 1 piezo speaker
- 5 LEDs
- 5 220 ohm resistors
- 1 TinkerKit wire
- 7 black jumper wires
- 8 colored jumper wires
Note: The potentiometer is introduced in Block 3. If you want to know more about how it works, read about it here.
Instructions
Code
Find the code in File>Examples>EducationShield>Block2-Sports>Projects>SimonSays
How it works
- The EducationShield library is included.
- The VU-meter variables are declared,
ledPins[]
,pinCount
andvuMeter
. - The Knob, Button and Melody objects are declared,
pot
,button
andpiezo
. - The game variables are declared to hold some game parameters,
turns_begin
,turns_max
,game
,turns[]
andblinkTime
. - In
setup()
, the VU-meter is configured and initialized. - The potentiometer is configured to have 5 levels.
- The button is initialized.
- The random number generator is initialized.
- In
loop()
, the program jumps to the functionnewGame()
. - In
newGame()
, all LEDs are blinked. - All LEDs are turned off.
- The program pauses for 500 milliseconds.
- A for loop loops for as many times as the value of
turns
. The first timenewGame()
is run,turns
is equal to 2. - In every loop of the for loop, a random number is generated between 0 and the value of
pinCount
, and stored ingame[]
. - The program jumps back to
loop()
. - The program jumps to the function
simonSays()
. - In
simonSays()
, a for loop loops for as many times as the value ofturns
. - In every loop of the for loop, an LED is turned on, on the position of the generated number stored in
game[]
. - The program pauses for as many milliseconds as the value of
blinkTime
. - The same LED is turned off.
- The program pauses again.
- The program jumps back to
loop()
. - The program pauses for 1000 milliseconds.
- The program jumps to the function
getInputs()
. - In
getInputs()
, a for loop loops as many times as the valueturns
. Each time the for loop runs, the following happens:- The variable
input
is declared. - A while loop loops as long as the button is not released in time. This means that the button is checked all the time but in short pulses, 10 milliseconds. This is to not completely stop the program and prevent it from checking the potentiometer.
- In the while loop, all LEDs are turned of.
- The LED on the position of the value read from the potentiometer, is turned on,
- When the button finally is released, the program leaves the while loop.
- The read value from the potentiometer is stored in
input
. - If input is equal to the current
game[]
value (if it is the first time the for loop runs, this means if input is equal togame[0]
), a scoring sound is played with the piezo. - The for loop continues and the next turn is checked.
- The variable
- When the for loop has looped through all the turns the program continues and pauses for 500 milliseconds.
- The program jumps to the function
levelUp()
. - In
levelUp()
, ifturns
is less thanturns_max
,turns
is increased with 1, making the game a little bit more difficult for the next round. - A winning sound is played with the piezo.
- The program jumps back to
getInputs()
. - The program jumps back to
loop()
. loop()
starts over and loops through the functions again.- In
getInputs()
, if input is not equal to the currentgame[]
value, the program jumps togameOver()
. - In
gameOver()
,turns
is set to be equal toturns_begin
again. - A game over sound is played with the piezo.
- The program jumps back to
getInputs()
. - The program leaves the
getInput()
function with the command return, and jumps back toloop()
. loop()
starts over and loops through the functions again.
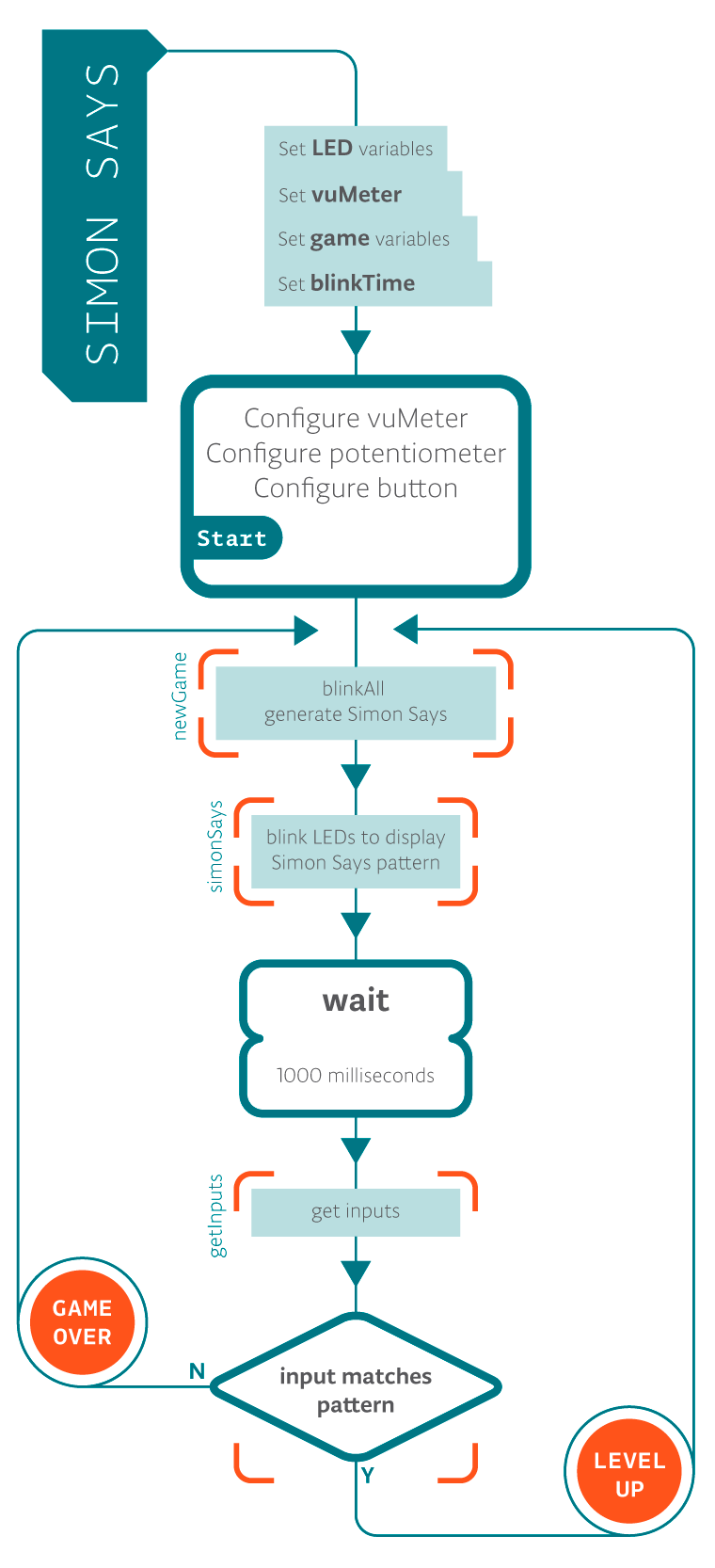
Troubleshooting
- Refer to the illustration and double check your connections. Make sure the shield and jumper wires are firmly connected. Check the LED polarities to make sure they are consistent and properly wired.
- If the button does not work, see reference for debugging buttons.
- If the VU-meter does not work correctly, see reference for debugging the VU-meter.
Learn by doing
- Make the game more challenging by making it go faster.
- Make it a two player game. Record the first players patterns and let the second player repeat it.